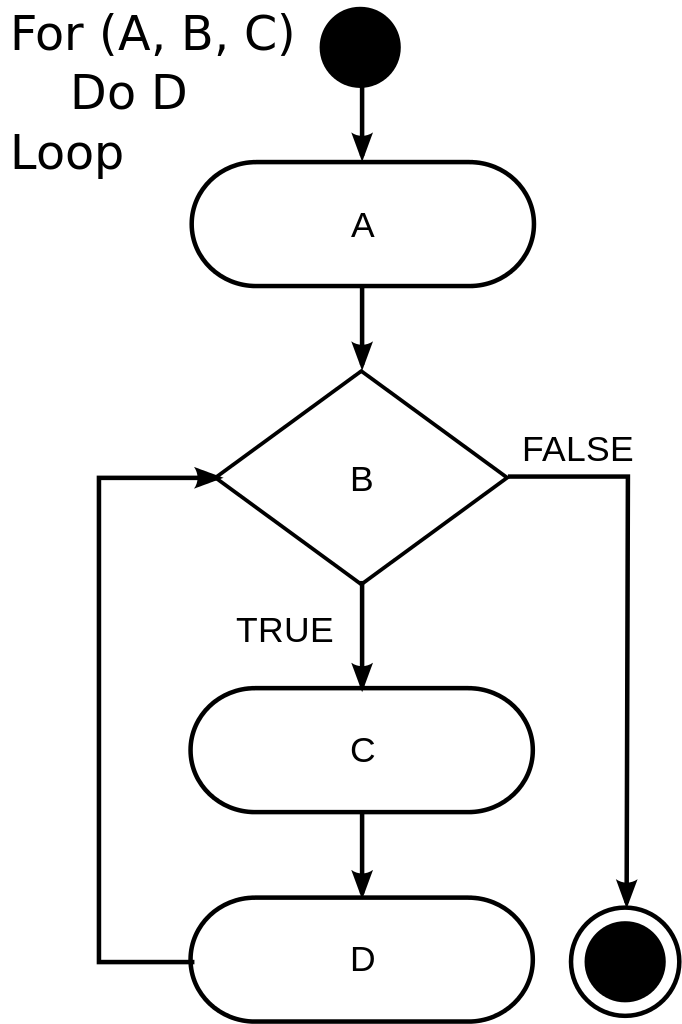
What are Loops in C++:-
Loop is the process of repetition of the certain steps until the given condition becomes false. They are always remains continue until the condition remains true. If the condition becomes false they will terminate.
Types of Loops:-
There are three types of loops in C++. These are while loop, Do while loop and For loop.
While loop in C++:-
It allows the program to repeat the collection of the statements enclosed in a body of a loop until the given condition becomes false.
Syntax of a C++ While Loop:-
While (condition)
{
Collection of Statements (the loops body)
}
Example of a While Loop:-
#include<iostream.h> #include<conio.h> main() { int a,b; cout<<" Input the first printing value :" ; cin>>a; cout<<endl; cout<<"Input the last printing value :" ; cin>>b; cout<<endl; while(a<=b) { cout<<" The Print out value is :"<<a; cout<<endl; a++; } getch(); }
Explanation:-
The above program will take the two values from the user. first value will be assigned to ‘a’ and second value will be assigned to ‘b’. Then the C++ while loop will come into the action and will check the condition if the condition is true then it will print ‘a’, after printing ‘a’ it will increment the value of ‘a’ and the control returned to it. Then it will terminate only if the given condition becomes false otherwise it will continue. The loop which has no terminating condition is called infinite loop.
Do While Loop in C++:-
It is used when user already does not know about the number of iterations. In this loop condition is written after the body of a loop. In this the body of a loop is executed at least once, even if the condition becomes false at the beginning.
Syntax of a C++ Do While Loop:-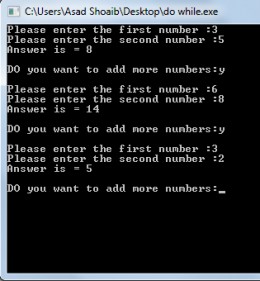
Do
{
Collection of statements
}
While (condition);
Example of a Do While Loop:-
#include<iostream.h> #include<conio.h> main() { int q,w; char z, ; do { cout<<"Please enter the first number :" ; cin>>q; cout<<"Please enter the second number :" ; cin>>w; cout<<"Answer is = " ; cout<<q+w<<endl; cout<<endl; cout<<"DO you want to add more numbers:" ; cin>>z; cout<<endl; } while(z=='y'); getch(); }
Explanation:-
The above program will take input two numbers, First number will be stored in a variable ‘q’ and the second number will be stored in a variable ‘w’. Then program will add the both numbers and show the result on the screen. After the result the program will ask user if he wanted to perform the operation again then press ‘y’. If the user entered ‘y’ then the control move above to the loop and all the statements will again executed but if the user entered any other character then it will terminate and the program will be close.
For Loop in C++:-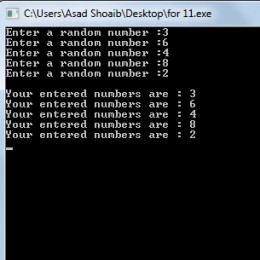
C++ For loop is known as the counter controlled loop. Unlike while and do while loop, for loop is given the specified number of iterations, This loop will continue until the maximum number of iteration given by the programmer completes or in other words condition becomes false.
Syntax of For Loop:-
For (starting value; given Condition; Increment in a starting value)
{
Collection of statements (The body)
}
Input and Output by using For Loop:-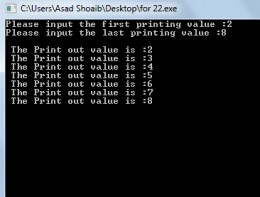
The code written below provides is an easy example of input and output of a For loop. It will give a good idea of how to use it.
The below written program will take 5 inputs in array named new with the help of For loop. After taking the input, another For loop will came into action and print the numbers into the screen which was assigned to array new.
#include<iostream.h> #include<conio.h> main() { int q,w; int new1[5]; for(q=0;q<5;q++) { cout<<"Enter a random number :"; cin>>new1[q]; } cout<<endl; for(w=0;w<5;w++) { cout<< "Your entered numbers are : "; cout<<new1[w]; cout<<endl; } getch(); }
Example of a For Loop:-
[yop_poll id=”4″] Here we will modify the code of an above program of a while loop to work for a For Loop.
Explanation:-
The below program will take the two values from the user. first value will be assigned to ‘j’ and second value will be assigned to ‘k’. Then, In a For loop the value of ‘j’ is assigned to the variable ‘m’ and the value of ‘m’ is lesser than the value of ‘k’ which shows that condition is true. Therefore the control will transfer within the body of a Loop, program will output the value of ‘m’ then it will increment it and the control will again transfer and the condition will check again this process will continue until the condition will be true else it will be terminate and the control moves out from the loop.
#include<iostream.h> #include<conio.h> main() { long j,k; cout<<"Input the first printing value :" ; cin>>j; cout<<"Input the last printing value :" ; cin>>k; cout<<endl; for(int m=a ; m<=b ; m++) { cout<<" The Print out value is :"<<m; cout<<endl; } getch(); }
If you like this article then provide us your opinions, your feedback is very appreciated. If you wanted to read my other articles then visit my homepage.
@ 2014 HellGeeks.com