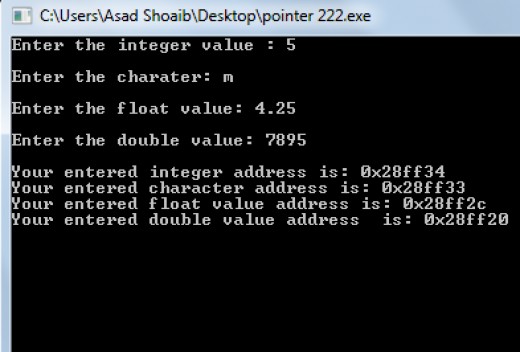
The pointers in C++ are simple variables which is declared by using the asterisk sign before the variable. It is used to store the memory addresses of data members and arrays of different data types. The reference operator is used to access the address of a variable which is assigned to the pointer.
Pointer Declaration in C++:-
The process of declaring pointers in C++ is same as we declare a simple variable but the asterisk sign is used to indicates that the declare variable is a pointer.
Syntax of a Pointer:-
The syntax for declaring C++ pointers is described below:
Data type *ptr;
Data type:-
It indicates towards the data type of a pointer.
Asterisk sign:-
This sign indicates that the declared variable is a pointer variable.
Ptr:-
It is the name of a pointer Variable.
Example:-
Int *ptr;
The above written statement indicates that the pointer named ptr is declared whose data type is integer which means it can only store the address of integer variable or integer array.
For Example:-
#include<iostream.h> #include<conio.h> #include<string.h> using namespace::std; main() { int *ptr; int q; cout<<"Enter any integer : "; cin>>q; cout<<endl; ptr=&q; cout<< "You entered the following integer : "<<*ptr<<endl<<endl; cout<<"whose address is : "<<ptr<<endl; getch(); }
Explanation:-
The above program will take input from the user and assigned it to the variable ‘q’ then assigned the address of ‘q’ to the pointer ptr. Then it will show the value of ‘q’ by using a pointer ptr and will also display its address.
The void Pointer:-
The type of pointers which can store the address of all types of data types is called Void pointer.
It is declared by the keyword void before the name of a pointer.
Example:
Void *ptr;
The above statement will declare the void pointer ptr which can store the address of all the variable of different data types.
Let’s consider the example program which will illustrate the use of the Void Pointer.
#include<iostream.h> #include<conio.h> #include<string.h> using namespace::std; main() { void *ptr1,*ptr2,*ptr3,*ptr4; int i; char c; float f; double d; cout<<"Enter the integer value : "; cin>>i; cout<<endl; cout<<"Enter the charater: "; cin>>c; cout<<endl; cout<<"Enter the float value: "; cin>>f; cout<<endl; cout<<"Enter the double value: "; cin>>d; cout<<endl; ptr1=&i; ptr2=&c; ptr3=&f; ptr4=&d; cout<<"Your entered integer address is: "<<ptr1<<endl; cout<<"Your entered character address is: "<<ptr2<<endl; cout<<"Your entered float value address is: "<<ptr3<<endl; cout<<"Your entered double value address is: "<<ptr4<<endl; getch(); }
Explanation:-
The above program will declare the four pointers of void type. Then it will declare the variable of integer, character, float and double type. Then it will assign values these variables according to their respective data types. Then the addresses of these variables will assign to the pointers ptr1, ptr2, ptr3 and ptr4 of void type. Then the program will display the address of these variables with the help of void type pointers.
Pointer initialization:-
The process in which pointer is assigned the memory address during declaration is called pointer initialization. The pointers in C++ should be initialized because if it does not then it could point towards something invalid. Pointer could also be initialized to Null or zero value.
Syntax of Pointer Initialization:-
Below written statement is the syntax of initializing the pointers.
Data type *ptr = &variable;
Example:
int q=80;
int *ptr = &q;
The above statement will declare a pointer “ptr” and it will assign the address of variable ‘q’ to “ptr” during initialization.
Below example will illustrate the process of pointer initialization.
#include<iostream.h> #include<conio.h> #include<string.h> using namespace::std; main() { int w; int *ptr = &w; cout<<" Enter any integer : "; cin>>*ptr; cout<<endl; cout<< "You entered the following integer : "<<*ptr<<endl<<endl; cout<<"whose address is : "<<ptr<<endl; getch(); }
Explanation:-
The above program will declare a variable ‘w’ then initialized the pointer ‘ptr’. Then it will show the entered value and address of ‘w’ with the help of pointer ‘ptr’.
Pointers and Arrays in C++:-
C++ Pointers are widely used with arrays. The name of the array represents the address of its first index, as all the elements of the arrays are stored in the consecutive memory locations, therefore pointers could access all the elements of the arrays by using its first index.
Example:-
Int set[8];
Int *ptr;
Ptr = set;
The above statements will declare the array ‘set’ and pointer ‘ptr’ and then it will assign the address of first index of the array ‘set’ to the pointer ‘ptr’.
Accessing Array Elements with Pointers:-
Array elements could be easily accessed by the pointers. The reference of pointer could be moved forward or backward by using the increment operator (++) or decrement operator (–). The elements present at address could be accessed by using a dereference operator (&).
Example:-
#include<iostream.h> #include<conio.h> #include<string.h> using namespace::std; main() { int set[5] = { 15,30,45,60,75}; int *ptr= set; cout<<*ptr<<endl; for(int q=1;q<5;q++) { cout<<*ptr++<<endl; } getch(); }
Explanation:-
The above program will assign the pointer to the array ‘set’, then it will display the value of its all the elements through pointer ‘ptr’ with the help of increment operator.
The array elements could also be accessed without moving the pointer reference permanently. The below example will demonstrate how to access the elements of array without moving the pointer reference permanently by using the pointers.
Example:-
#include<iostream.h> #include<conio.h> #include<string.h> using namespace::std; main() { int set[5] = { 15,30,45,60,75}; int *ptr= set; cout<<*ptr<<endl; cout<<*(ptr+1)<<endl; cout<<*(ptr+2)<<endl; cout<<*(ptr+3)<<endl; cout<<*(ptr+4)<<endl; getch(); }
[yop_poll id=”11″] If you like my article on pointers in C++ and wanted to read my other articles then visit my Homepage.
@ 2014 HellGeeks.com