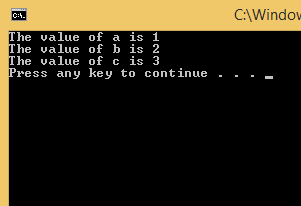
In programming, the multilevel inheritance is a technique or process in which a child class is inherited from another derived class. Let’s think of it in terms of a family tree. We have a class father, Son class is inherited from father class and grandson class is inherited from Son class. Therefore, grandson class will have all the properties and functionality possessed by father class and son class combined.
For Example:-
#include<iostream> #include<conio.h> class Father { protected: int a; public: Father() { a = 5; }; }; class Son : public Father { protected: int b; public: Son() : Father() { b = 9; }; }; class GrandSon : public Son { protected: int c; public: GrandSon() :Son() { c = 0; c = a + b; } void Show() { std::cout << " a + b = " << c<<"\n"<<"\n"<<"\n"; } }; main() { GrandSon obj; obj.Show(); getch(); }
Explanation:-
In above program we have declared three classes Father class, Son class and Grandson class. Father class have protected variable ‘a’ which is inherited in Son class, therefore Son class have two protected data members ‘a’ and ‘b’, GrandSon class has three data members ‘a’, ‘b’ and ‘c’. ‘a’ and ‘b’ is inherited and ‘c’ is its own data member. In a main function we create a GrandSon object and call function “Show()” which will add the inherited members of GrandSon class.
Multilevel Inheritance with Parameters:-
In multilevel inheritance, the parameter could be passed from Child to its Father and from Father to its GrandFather. Lets take a look towards an example, which will elaborate the working of multilevel inheritance.
Example:-
#include<iostream> #include<conio.h> class Father { private: int a; public: void set(int x) { a = x; } void out() { std::cout << "The value of a is " << a << std::endl; } }; class Son : public Father { private: int b; public: void set(int m, int n) { Father::set(m); b = n; } void out() { Father::out(); std::cout << "The value of b is " << b << std::endl; } }; class GrandSon : public Son { private: int c; public: void set(int g, int h, int k) { Son::set(g, h); c = k; } void out() { Son::out(); std::cout << "The value of c is " << c << std::endl; } }; main() { GrandSon obj; obj.set(1, 2, 3); obj.out(); getch(); }
Explanation:-
In above example we are transferring parameters from a Grandson object to its Father and then to its GrandFather. In multilevel inheritance, if child and its parent has functions of same name then function of child will be executed.
Nice example….