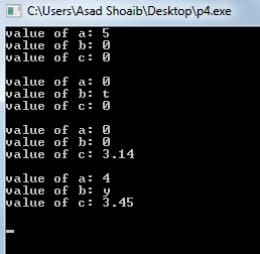
In this article we will review types of Constructors in C++, types of copy constructors and difference between shallow and deep copy.
Constructors in C++:-
In object oriented languages, constructors are basically a special kind of member functions that are automatically executed when the object of that class is created, they have the same name as the name of a class, there could be multiple constructors defined in a class which is known as constructor overloading. We will explain later in this article that how overloaded constructors are used in C++.
Depending on the requirements of the program constructors could be used to perform the functionality like normal member functions, but in normal routine they are used to initialize the data members or attributes of a class, one thing should be remembered that the constructors could not return any value therefore there return type is always void.
Syntax
The syntax of declaring the constructor of a class Name is as follows:
Name()
{
Constructor body
}
. It doesn’t matter, if you have written void before the constructor, it will still not generate an error.
Name: It indicates towards the name of the constructor. The name must be same as the name of the class in which the constructor is declared.
For Example:-
#include<iostream.h> #include<conio.h> class comp { private: int model; public: comp() { cout<<"Object of Computer is created.... :"<<endl; } }; main() { comp x,y,z; getch(); }
Explanation:-
The above program will declare a class comp and then it uses a simple output statement in a constructor, which is automatically executed, when the object of comp is created.
Passing parameters to Constructors in C++:-
The process of passing parameters to the constructor are same as we pass the parameters to the simple member functions but with a little difference, which is that they accept parameters at the time of a declaration of the object of a Class. The parameters are written in parenthesis along with the object name in the declaration statement.
Syntax of Passing parameters to constructors in C++:-
Following is the syntax of passing parameters to constructors:-
Type object_name(parameters);
Type: It indicates towards the name of a class whose object is to be created.
Object_name: It indicates towards the name of the object to be created.
Parameters: It indicates the list of parameters passed to the constructor, it could be one or more, depending upon the signatures of the constructor.
For Example:-
#include<iostream.h> #include<conio.h> class Student { private: int marks; char grade; public: Student(int m, char g) { marks= m; grade= g; } void show() { cout<<"Marks ="<<marks<<endl; cout<<"Grade = "<<grade<<endl; } }; main() { Student s1(730, 'A'), s2(621,'B'); cout<<"Record of student 1:"<<endl; s1.show(); cout<<"Record of student 2:"<<endl; s2.show(); getch(); }
Explanation:-
The above program will declare a class Student with two data members named marks and grade. It will then initialize the data members in the constructor of a class. One more function is created to show the information about a student.
In the Main function the two objects of a Student is created whom we assigned the values at the time of declaration according to the signatures of their respected constructor. Then it will call the show function through objects to display attributes of the objects.
Types of Constructors in C++:-
There are two types of constructors in C++.
1. Default constructors.
2. Overloaded Constructor.
Default Constructor in C++:-
If the programmer does not write a constructor in a Class then the compiler will automatically generate a default parameter less constructor which will initialize the fields of a Class.
If the programmer defined the parameterize constructor, then the compiler will not generate the default constructor of a Class, Therefore user have to explicitly write the parameter less constructor.
For Example:-
#include<iostream.h> #include<conio.h> class test { private: int a; int b; public: test() { a= 0; b= 0; } void show() { cout<<"value of a: "<<a<<endl; cout<<"value of b: "<<b<<endl; } }; main() { test q1; q1.show(); getch(); }
Explanation:-
As you can see we didn’t write any constructor, therefore the compiler will generate one by default which will initialize the fields to zero.
Overloaded Constructor in C++:-
It is the process of declaring multiple constructors with the same name but different parameters is known as constructor overloading. In the same Class we could declare multiple Constructors but they should differ in number of parameters, sequence and type of signatures.
For Example:-
#include<iostream.h> #include<conio.h> class test { private: int a; char b; double c; public: test(int q) { a=q; b= '0'; c=0; } test(char q) { a=0; b= q; c=0; } test(double q) { a=0; b='0'; c=q; } test(int q1, char q2, double q3) { a=q1; b=q2; c=q3; } void show() { cout<<"value of a: "<<a<<endl; cout<<"value of b: "<<b<<endl; cout<<"value of c: "<<c<<endl<<endl; } }; main() { test q1(5); test q2('t'); test q3(3.14); test q4(4, 'y', 3.45); q1.show(); q2.show(); q3.show(); q4.show(); getch(); }
Explanation:-
The above program created an objects and pass parameters at the time of creation according to the signatures of the respective constructors defined in a class.
Types of Copy Constructors in C++:-
1. Default copy constructor.
2. Shallow copy constructor.
Default copy constructor in C++:-
It is a type of a copy constructor which is used to initialize the newly created object with the previously created object of a same type is called default copy constructor. The objects are assigned by using the assignment operator or by giving object as a parameter. It will copy the values of fields.
Syntax
The syntax of using default copy constructor is as follows:
Class_name new_object(previous_object);
Or
Class_name new_object = previous_object;
Class_name: It is the name of a class and indicates towards the type of object to be created.
New_object: It indicates the name of the object to be created.
Previous_object: It indicates towards the same type of object whose values to be copied.
Example:-
#include<iostream.h> #include<conio.h> #include<string.h> class Book { private: int pages; char title[3]; public: Book(int q, char w[3]) { pages= q; for(int i=0 ; i<3 ; i++) { title[i]= w[i]; } } void show() { cout<<"Title:"<<title<<endl; cout<<"Pages:"<<pages<<endl<<endl; } }; main() { Book b1(25, "C++"); Book b2(b1); Book b3= b1; cout<<"detail of b1:"<<endl; b1.show(); cout<<"detail of b2:"<<endl; b2.show(); cout<<"detail of b3:"<<endl; b3.show(); getch(); }
Explanation:-
The above program will declare an object b1 and entered the parameters at runtime according to the constructor. Then another object b2 is declared which took b1 as a parameter, another object b3 is declared which we assigned b1. Then the values of the attributes of b1 will be assigned to b2 and b3. At the end, the details of objects b1, b2 and b3 will be displayed which are the same.
Difference between Shallow and Deep copy Constructor in C++:-
In case of dynamic memory allocation,the major difference in both copy constructors is that when we write shallow copy we assign the addresses of pointers to each other therefore they points towards the same memory location. When we write deep copy we make a new memory location in the heap and assign pointers the values instead of an address. Below examples will completely clarify the difference between shallow and deep copy in c++.
Shallow Copy Constructor in C++:-
Shallow copy constructor is used when the dynamic memory allocation is not used in classes. The compiler generates shallow copy by default. Class (class &obj) this is the syntax of writing a copy constructor manually.
Following example will show how to write this constructor manually.
For example:-
#include<iostream.h> #include<conio.h> class test { public: int *a; public: test(int q) { a= new int; *a=q; } test(test &obj) { a=obj.a; } void mod(int e) { *a=e; } void show() { cout<<"The value is :"<<*a<<endl; } }; main() { test q1(8); test q2= q1; q2.show(); q1.mod(5); q2.show(); getch(); }
Explanation:-
In the above code we have used dynamic memory allocation and performed the shallow copy in the default constructor. If we do not write it, then compiler will generate by default the shallow copy.
As you can see the pointer a both objects q1 and q2 points towards the same memory location in a heap, therefore when we modify the value of the object q1 the value of q2 will be modified that means both q1 and q2 points towards a same value.
Deep copy constructor in C++:-
When we have to deal with the dynamic memory allocation in classes, then we should always use a deep copy.
Following code will illustrate the use of deep copy.
Example:-
#include<iostream.h> #include<conio.h> class test { public: int *a; public: test(int q) { a= new int; *a=q; } test(test &obj) { a= new int; *a= *obj.a; } void mod(int e) { *a=e; } void show() { cout<<"The value is :"<<*a<<endl; } }; main() { test q1(8); test q2= q1; q2.show(); q1.mod(5); q2.show(); getch(); }
Explanation:-
As you can see, the only difference between this example and shallow copy example is that we created a new integer type in a heap and assigned its address to object q2. Then we assigned the value of pointers to each other. One thing should be remembered in shallow copy we assign the address of pointers to each other but in deep copy we assign the values.
Destructors in C++:-
These are the type of member function which are automatically executed when an object of that class is destroyed is called a destructor. The destructor has no return type and its name is same as class name, it cannot accept any parameters. The tilde sign(~) is written before the destructor name.
Syntax of a Destructor in C++:-
The Syntax of declaring destructor is as follows:
~name()
{
Destructor body
}
~name: It shows the name of a destructor.
Example:-
#include<iostream.h> #include<conio.h> class test { private: int a; public: test() { cout<<"object is created :"<<endl<<endl; } ~test() { cout<<"Object is destroyed"<<endl<<endl; } }; main() { test *p1= new test; test *p2= new test; delete p1; delete p2; getch(); }
Explanation:-
The above program will declare two objects of a Class test, therefore, its constructor is called and “object is created” will be displayed twice, then at the termination the destructor will call and “object is destroyed” will be printed.
[yop_poll id=”16″] If you like my article on constructors and destructors in C++ and wanted to read my other articles then visit my Homepage.
@ 2014 HellGeeks.com